Authentication
OAuth Providers
OAuth providers allow users to sign in to your application using their existing accounts from popular services like Google or Facebook. By integrating OAuth providers, you can provide a seamless and secure login experience for your users.
Integrating OAuth Providers
To integrate an OAuth provider in your application, follow these steps:
Register with the provider
- Go to the developer console of the OAuth provider (e.g., Google Developers Console).
- Create a new project and configure the OAuth settings.
- Set the return URL for your application to
https://your.app/api/oauth/providerId/callback
, replacingyour.app
with your domain andproviderId
with the ID of the OAuth provider (e.g.,google
). - Obtain the client ID and client secret for your application.
Configure the provider in your application
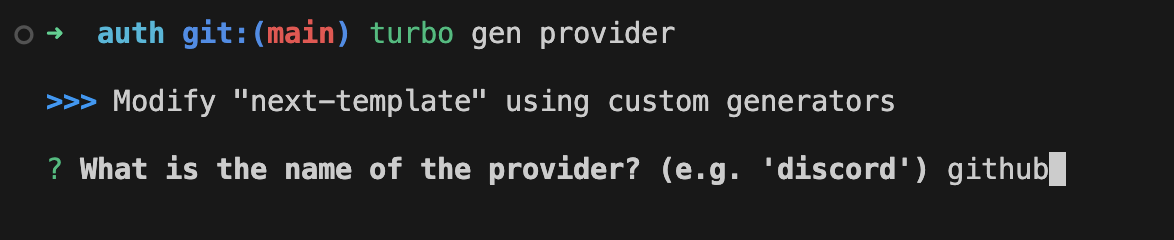
- Add the client ID and client secret to your environment variables.
- Generate a basic OAuth provider integration using a generator:
turbo gen provider
- The generator will prompt you to select the OAuth provider you want to integrate (e.g., GitHub, Discord).
- Write the logic to handle the OAuth flow in your application.
Enabling OAuth Providers
To enable OAuth providers in your application, you need to configure the provider settings and add them to the list of available providers in packages/auth/src/providers/index.ts
. Here is an example of how to enable Google and Facebook OAuth providers:
import { FACEBOOK_PROVIDER_ID, provider as facebook } from './facebook'
import { GOOGLE_PROVIDER_ID, provider as google } from './google'
/**
* List of OAuth providers, keyed by provider ID.
* Add or remove providers here to enable or disable them.
*/
const providers: Record<string, OAuthProvider> = {
[GOOGLE_PROVIDER_ID]: google,
[FACEBOOK_PROVIDER_ID]: facebook,
}